Implementing Ollama in Google Colab: Building a Local RAG Pipeline with DeepSeek-R1 1.5B, LangChain, FAISS, and ChromaDB for Q&A
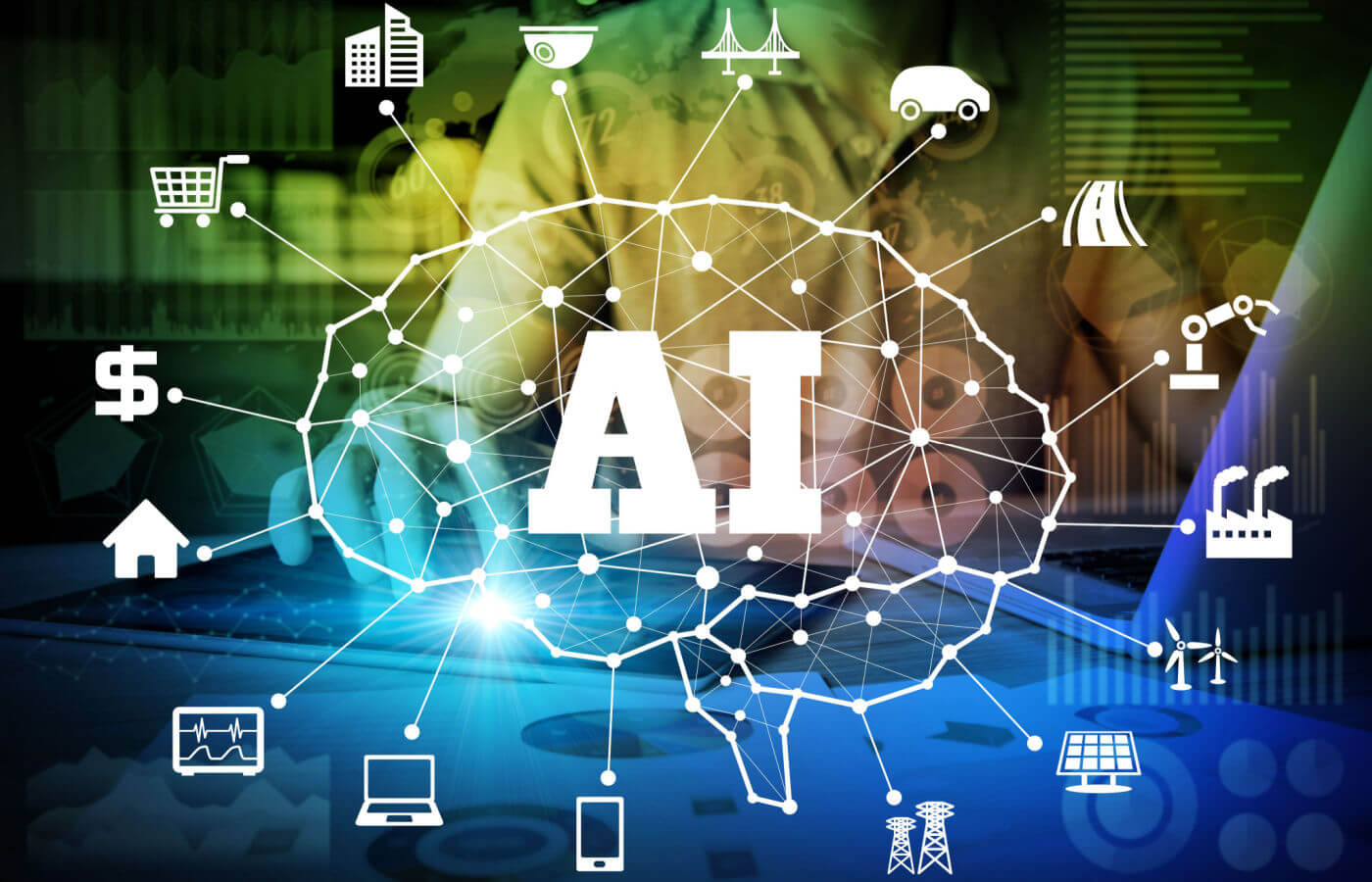
Building a Local Retrieval-Augmented Generation (RAG) Pipeline Using Ollama and Google Colab
With the evolution of artificial intelligence, tools that facilitate the development of machine learning applications have become increasingly accessible. One such compelling combination is the ability to implement a Local Retrieval-Augmented Generation (RAG) pipeline using technologies like Ollama, LangChain, FAISS, and ChromaDB. This guide aims to explain how to set up this pipeline in Google Colab, focusing on a model like DeepSeek-R1 1.5B.
What is a Local RAG Pipeline?
Understanding the Components
- Ollama: A tool used for deploying and running large language models efficiently.
- LangChain: An adaptable framework designed for building applications powered by language models.
- FAISS: Facebook AI Similarity Search, a library for efficient similarity search and clustering of dense vectors.
- ChromaDB: A document management system optimized for embedding and search, enhancing the capabilities of RAG pipelines.
- DeepSeek-R1 1.5B: A large language model engineered for generative tasks, providing robust question and answer features.
How RAG Works
A RAG model combines traditional retrieval techniques with generation capabilities. It retrieves relevant information from a dataset using an index and then augments existing queries by generating text based on that retrieved data. This provides more accurate and contextually relevant responses.
Setting Up Your Environment
Requirements
To successfully build a Local RAG pipeline, you’ll need:
- A Google account for Google Colab access.
- Basic familiarity with Python programming.
- Libraries for machine learning: TensorFlow, LangChain, FAISS, and ChromaDB.
Installation Steps
Access Google Colab:
- Navigate to Google Colab and create a new notebook.
- Install Necessary Libraries:
Open a code cell and install the required libraries using pip:!pip install langchain faiss-cpu chromadb
Implementing the Pipeline
Loading the Model
Load the DeepSeek-R1 1.5B model using Ollama. Ensure that you have the model downloaded and available in your environment.
from ollama import Ollama
model = Ollama("DeepSeek-R1 1.5B")
Setting Up LangChain
LangChain helps in managing how you interact with the model and integrate various components. You need to initialize LangChain to utilize it for query generation and response handling.
from langchain import LanguageChain
chain = LanguageChain(model=model)
Utilizing FAISS for Vector Search
To enable efficient searching, we can use FAISS to create an index for the embeddings derived from your dataset.
import faiss
import numpy as np
# Create some example vectors
data = np.random.rand(1000, 768).astype('float32') # Assuming each embedding is of size 768
index = faiss.IndexFlatL2(768)
index.add(data)
Integrating ChromaDB
ChromaDB serves as your document manager where you store embeddings and interactively search for relevant content. Ensure that it is initialized correctly.
from chromadb import Client
chroma_client = Client()
Searching for Relevant Content
Once everything is set up, you can run a query. The system first retrieves relevant data via FAISS, and then generates a response using DeepSeek-R1.
query = "What are the main applications of AI?"
# Use FAISS to find the closest embeddings
D, I = index.search(query_embedding, k=5)
# Generate answer using LangChain
response = chain.run(query)
Testing Your Pipeline
After implementing the above code snippets, run some test queries against your RAG pipeline. This helps ensure everything is functioning correctly. Check both the accuracy and relevance of the responses generated to verify the setup meets your expectations.
By following these steps, you can set up a comprehensive Local RAG pipeline using leading-edge technologies designed for efficient AI applications. Embracing this approach will enable you to access advanced generative capabilities for various tasks, including question and answer scenarios.