Overview of the OpenAI Agents SDK and Responses API
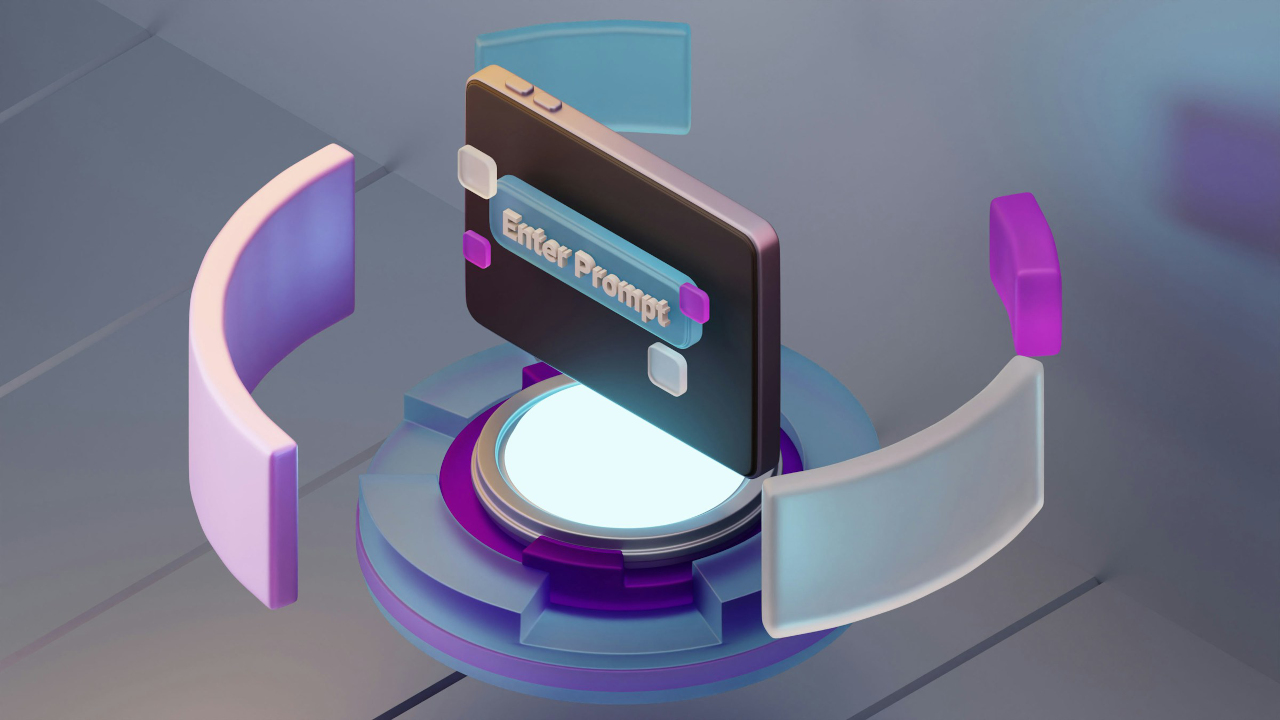
Introduction to OpenAI’s Agents SDK
OpenAI has rolled out its Agents SDK, which aims to streamline interactions with artificial intelligence by enabling easier orchestration of multiple processes. The previous methods of using AI agents often involved intricate programming and extensive prompt iterations, creating challenges for developers. OpenAI acknowledges this complexity and is now introducing a simpler framework to manage agent interactions effectively.
Understanding the Challenges with AI Agents
AI agent tasks typically require multiple processes to work in coordination. For instance, one task may initiate another, with results flowing back to a final process. Ensuring that outputs are returned in a standardized format—such as text, files, or images—can be quite challenging.
Key Issues in Agent Orchestration
- Independent Processes: Agents often operate separately, making it hard to achieve synchronized outcomes.
- Format Consistency: Outputs must adhere to an expected format, complicating integrations.
- Error Handling: Managing and explaining errors can be cumbersome, adding to the complexities of orchestration.
- Token Management: Utilizing large language models (LLMs) requires careful token management, as tokens are crucial for various functionalities.
Due to these complications, the orchestration of AI agents remains an unsolved challenge in the tech industry.
New Features in OpenAI’s SDK
To simplify the orchestration process, OpenAI has introduced new APIs, notably the Responses API, which addresses some underlying assumptions that were previously challenges when utilizing chat agents.
Example of the Responses API
Using the new API, developers can easily capture outputs. Here’s a basic example:
from openai import OpenAI
client = OpenAI()
response = client.responses.create(
model="gpt-4o",
input="Write a one-sentence bedtime story about a unicorn."
)
print(response.output_text)
Exploring New Tools and Features
OpenAI’s SDK comes with new tools that enhance agent capabilities.
Web Search Tool
This feature allows an agent to perform web searches for real-time information. Here’s how it can be implemented:
from openai import OpenAI
client = OpenAI()
response = client.responses.create(
model="gpt-4o",
tools=[{"type": "web_search_preview"}],
input="What Kubernetes news story appeared today?"
)
print(response.output_text)
The output will include references to cited articles, allowing for time- or location-based queries.
File Search Tool
The File Search tool acts as a hosted vector store. Below is an example of how to use it:
from openai import OpenAI
client = OpenAI()
response = client.responses.create(
model="gpt-4o-mini",
input="What is deep research by OpenAI?",
tools=[{
"type": "file_search",
"vector_store_ids": [""]
}]
)
print(response)
Computer Use Tool
Another unique feature is the Computer Use Tool, which allows an agent to execute actions on a computer continuously. It can perform tasks like simulating clicks or typing text, returning screenshots of the outcomes, reflecting a step towards automation in broader contexts.
Practical Implementation of Agents
Let’s delve into how to use these agents practically. Begin by ensuring Python and necessary packages are installed. You can set your OpenAI API key and check basic functionality with a sample code snippet:
from agents import Agent, Runner
agent = Agent(name="Assistant", instructions="You are a helpful assistant")
result = Runner.run_sync(agent, "Write a haiku about recursion in programming.")
print(result.final_output)
This will trigger an agent and showcase its capability in generating structured content.
Creating Nested Agents
The SDK also allows for nesting agents, enabling complex interactions. For example, you could set up language-specific agents for a multi-lingual operational scenario. Here’s how you would implement basic multi-lingual support:
from agents import Agent, Runner
import asyncio
spanish_agent = Agent(
name="Spanish agent",
instructions="You only speak Spanish."
)
english_agent = Agent(
name="English agent",
instructions="You only speak English."
)
triage_agent = Agent(
name="Triage agent",
instructions="Handoff to the appropriate agent based on the language of the request.",
handoffs=[spanish_agent, english_agent],
)
async def main():
result = await Runner.run(triage_agent, input="Hola, ¿cómo estás?")
print(result.final_output)
if __name__ == "__main__":
asyncio.run(main())
Enhancing Language Capabilities
By introducing a third language (e.g., German), you can bolster the triage agent’s capabilities, ensuring that requests in multiple languages are handled correctly. This adaptability highlights how agents can work together to provide comprehensive responses.
By leveraging these new features, OpenAI aims to create a more intuitive and user-friendly environment for developers looking to harness the full potential of AI orchestration.